The first code snippet I’d like to share comes from a simple game I created for my daughter. The origin is a challenge from 100 days of SwiftUI, where you are supposed to create an app for training the times tables. Back then I only got the logic working, but when she started with multiplications in school this year, she asked if could finish it for her.
At first I planned to get it on her phone with Test Flight, but it turns out that you have to be sixteen to install Test Flight. So I realised the only viable option was to go through App Store, and since that meant the app would be available to anyone with an iPhone I’d better put some effort in the interface.
With some input from my target audience I ended up with a gradient based theme, and now we’ve arrived to the reason for this post, the progress tracking view.
It’s a pretty simple table layout (or a LazyVgrid to be accurate) that shows how many times you’ve played – or practiced – each table, that is represented by different gradients, looking a bit like candy. Maybe that’s why my daughter likes them…
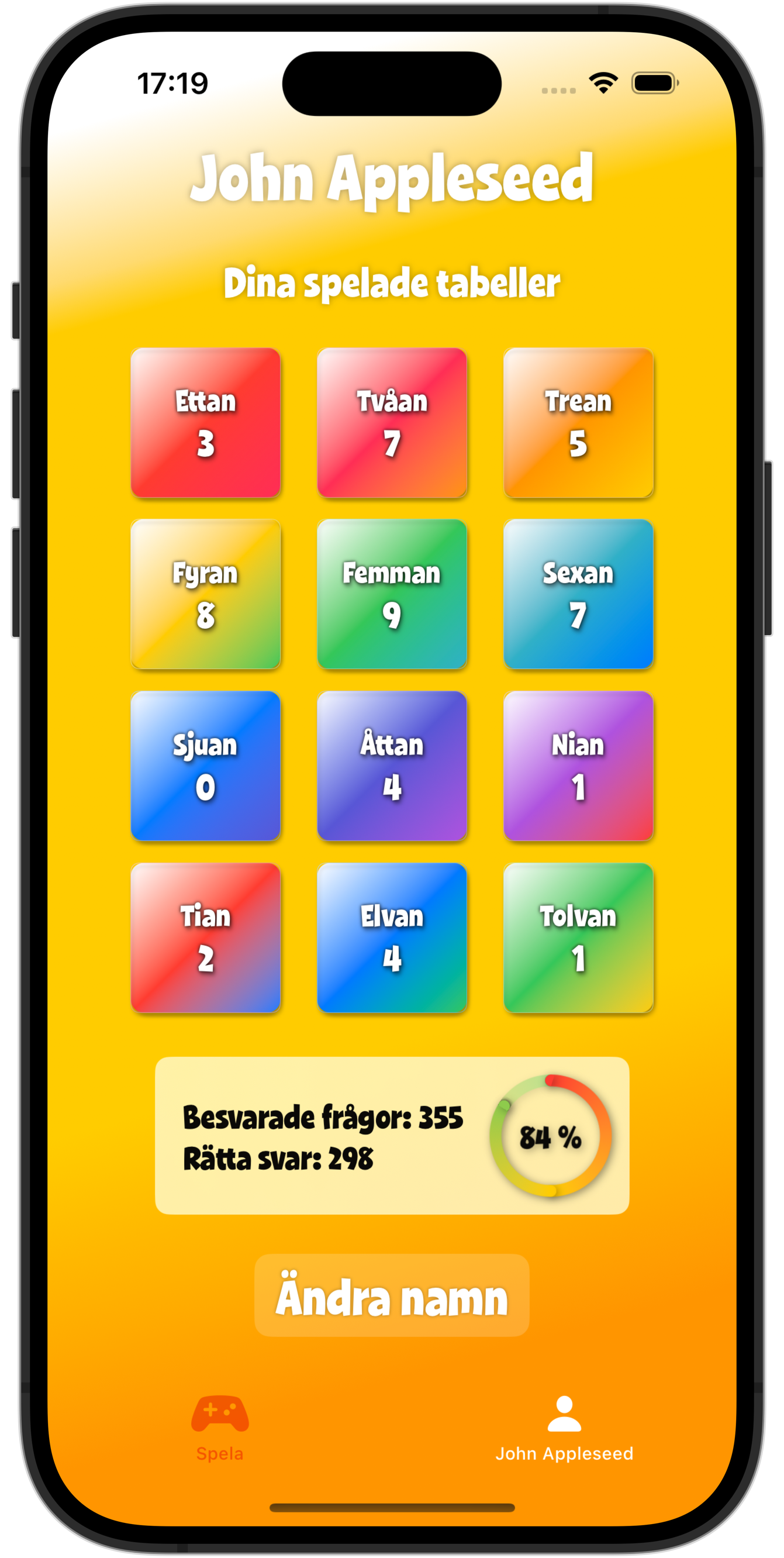
The code is pretty straightforward. Each table is created by calling a GradientView that takes a few arguments.
struct GradientView: View {
let gradient: Gradient
let width: CGFloat
let height: CGFloat
let radius: CGFloat
let tabell: LocalizedStringResource
let spel: Int
var body: some View {
LinearGradient(gradient: gradient, startPoint: .topLeading, endPoint: .bottomTrailing)
.clipShape(RoundedRectangle(cornerRadius: radius))
.frame(width: width, height: height, alignment: .center)
.overlay {
RoundedRectangle(cornerRadius: 5)
.strokeBorder(.ultraThinMaterial, lineWidth: 0.35)
VStack {
Text(tabell)
.font(Font.custom(Fonts.primary, size: 22))
Text("\(spel)")
.font(Font.custom(Fonts.primary, size: 28))
}
.shadow(color: .black, radius: 1)
}
.shadow(radius: 1, x: 1, y: 2)
.foregroundStyle(.white)
}
}
The only important properties to create the View are gradient, width, height and radius. The last two are used to pass in the text that is displayed and can be replaced with whatever fits your needs.
Then I simply call the view in a ForEach, inside my LazyVGrid.
LazyVGrid(columns: gridItems, spacing: width / 32) {
ForEach(gameController.player.timesEachTablePlayed.indices.dropFirst(), id: \.self) { i in
GradientView(
gradient: Gradients.gradients[i],
width: height / 8,
height: height / 8,
radius: 8,
tabell: globals.tabellerna[i],
spel: gameController.player.timesEachTablePlayed[i]
)
}
}
There are a few things worth mentioning. First, I’ve created an array with predefined gradients to match the twelve playable times tables.
struct Gradients {
static let gradients: [Gradient] = [
Gradient(colors: [Color(.blue), Color(.yellow), Color(.red)]),
Gradient(colors: [Color(.white), Color(.red), Color(.pink)]),
Gradient(colors: [Color(.white), Color(.pink), Color(.orange)]),
... and so on ...
]
}
Second, the main view is wrapped in a Geometry Reader to make the layout automatically adjust to the current screen size, hence height/8
, being passed to the GradientView. Since I want my buttons to be squares, I don’t bother using height and instead pass width for both properties.
I admit that this solution has a serious problem. If you can’t be sure that your ForEach will return the same number of indices on each run, using a fixed set of pre defined gradients is probably not good idea, unless you want your app to crash. But an easy fix could be to use the modulo operator and have the gradients repeat when you run out of them.
I admit that you could probably find a smarter solution, but if want to become a contributor to the swift community, I’ll have to start somewhere.
This is somewhere.